Below is a PowerShell script that reads user information from a CSV file and sets the account expiration date to two days from the current date for each user in Active Directory. Please make sure to adjust the CSV file structure according to your needs:
CSV structure:
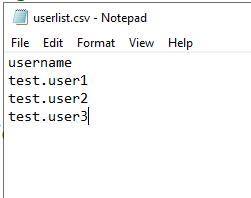
# Import the Active Directory module
Import-Module ActiveDirectory
# Specify the path to the CSV file containing user information
$csvFilePath = "C:\Path\To\Your\Users.csv"
# Read user information from the CSV file
$userData = Import-Csv $csvFilePath
# Function to set account expiration date for AD user
function Set-ADUserExpiryDate {
param (
[string]$username,
[int]$daysToAdd
)
$user = Get-ADUser -Identity $username
if ($user) {
$expiryDate = (Get-Date).AddDays($daysToAdd)
Set-ADAccountExpiration -Identity $username -DateTime $expiryDate
Write-Host "Account expiration date set for $username: $expiryDate"
} else {
Write-Host "User $username not found in Active Directory."
}
}
# Loop through each user in the CSV and set the expiration date
foreach ($user in $userData) {
Set-ADUserExpiryDate -username $user.Username -daysToAdd 2
}
Please ensure that your CSV file (Users.csv) has a header row with the column Username (case-sensitive) containing the usernames for which you want to set the expiration date.
Note: Make sure to test scripts in a safe environment before applying them to a production Active Directory. Ensure that you have the necessary permissions to modify user account information.